SVG Vector graphics with Snap.svg
March 16, 2017
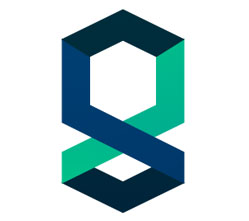
Preface
Snap.svg “The JavaScript SVG library for the modern web” is an all in one javascript library for working with vector based graphics, the documentation is a bit sparse, and even though I’ve worked with Snap.svg in the past, I would like to make a getting started post for future reference and to help those just getting started, at the end of these code samples both you and I will be able to present and animate with vector graphics, so lets get started.
Hey,( totally optional) if you are new to SVG Graphics, you might like this other post:
Let's start by setting up - Basics
Step 1: Import Snap.svg and create a simple vector Graphic
See the Pen Snap.svg Setup by Eugenio Keno Leon (@k3no) on CodePen.
Explanation:
- After importing Snap.svg (check the JS tab) you will then need an SVG element in your HTML, you will also need to position it and size it, I am using Bootstrap to simplify things, but you can use something else (plain CSS,other frameworks, JS).
- Once you have your SVG element, reference it in Javascript and that's it, you can start adding elements,(circles in this case and changing their appearance).
You try it. Recreate the previous code sample with Rectangles and different colors
Step 2: Animate a simple shape with Snap.svg
See the Pen Snap.svg Animation by Eugenio Keno Leon (@k3no) on CodePen.
Explanation: Snap provides a handy animate() function for your basic animation needs, it is a simple affair where you provide the attributes to change ( from their current value), time to make those changes, easing function (rate of change function) and a callback function to tie animations or call other functions.
You try it. Recreate the previous code sample with multiple elements and vertical animations
Step 3: Add mouse events & Touch Events
See the Pen Snap.svg Interaction by Eugenio Keno Leon (@k3no) on CodePen.
Explanation: Like with animations, Snap tries to make it super simple to add interactions like mouse and touch events by simply calling the relevant method on your snap element, something like:
YOUR-SNAP-ELEMENT.mouseup(FUNCTION TO HANDLE MOUSEUP)
And you are done : )
Note: I had some issues with the stock drag function, since it doesn't play nice with responsive displays, so some extra calculations need to be made for it to work, check the code.
You try it. Add interaction to multiple snap elements
Intermediate Snap.svg
Importing vector graphics
Beyond simple primitives made with Snap, you can also import vector art made on other programs:Inkscape (Free) and Illustrator (Paid) are popular options , let’s figure out how to import some art…
Step 4: Import some vector art !
See the Pen Snap.svg Loading external svgs by Eugenio Keno Leon (@k3no) on CodePen.
Explanation:
Importing external SVG Artwork is done by using snaps
load function and a callback function to deal with the result. Note that while you can display complex art relatively easy, if you want to deal with parts of your svg programatically you need to format your svgs into something snap can work with, in this case my original svg artwork was an Illustrator file that got converted into a compound path
(Object->CompoundPath->make) and named as "mrCube" in the layer option, and then saved as an svg. This way you can select and modify it once the svg is loaded with
geom = data.select("#mrCube")
You try it. Make and import an external vector graphic.
In order to make more complex and interactive graphics, we need to deal with multiple elements and complex paths, so let’s try that.
Step 5: Import a complex svg with multiple elements and interact with it via snap.
See the Pen Snap.svg Loading Complex Svgs by Eugenio Keno Leon (@k3no) on CodePen.
Explanation: A lot is happening here, so let's go step by step:
- we are loading an external svg like before, but in this case the svg has 2 named paths truckY and textY, we then select each one by id with data.select("#id"), like a css selector.
- We now can move and resize them with by defining a Matrix (A coordinate system, you can read more about it here: Matrix transformations )
- Next we are attaching a hover to the truck and animating the text inside the Hover Handler
You try it. Make and import an external vector graphic with multiple elements and reference & modify them in snap
Note: Our animations now use
mina easing, to give the text that bounce, there is also an abbreviated transform animation that confusingly is not explained in Snaps documentation, but it is in Raphaël, a very similar svg library from the same author =>
Raphaël Library element transform.
All together now<
So now that we have a basic understanding of Snap.svg let’s try making an interactive animated vector graphic with Snap !
See the Pen Snap.svg Demo wip by Eugenio Keno Leon (@k3no) on CodePen.
Explanation: If you look under the hood( pun intended ), you might find there is a lot of code, but there is also a lot of repetition and there is nothing new from the previous examples, so nothing too scary; in general it goes like this:
- Load and create all the separate elements and their initial attributes like position and visibility
- Attach interaction events and call animations
- The remaining code is just animation functions and event handlers
You try it. Create and animate multiple Svg elements and add interaction to a couple of them.
Conclusion
I personally love working with SVGs, they scale and display nicely and there are great tools for creating them, the problem usually is that they are not as performant as say HTML5 Canvas or CSS or easy to work with, Snap.svg is a super cool library that tries to bridge this gap and provide a basic yet complete set of tools for working with svg on the web, while not a complete replacement or solution,you can add very cool interactions in no time ( once you follow this or other guides ).
I hope this code samples spark your interest in making some cool interactive svg graphics for the web.
Best,
Keno